Linked list Interview Question

Compare two linked lists
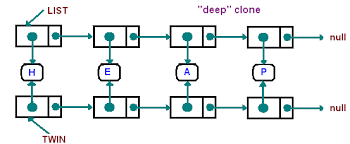
You’re given the pointer to the head nodes of two linked lists. Compare the data in the nodes of the linked lists to check if they are equal. The lists are equal only if they have the same number of nodes and corresponding nodes contain the same data. Either head pointer given may be null meaning that the corresponding list is empty.
Input FormatYou have to complete the int CompareLists(Node* headA, Node* headB) method which takes two arguments - the heads of the two linked lists to compare. You should NOT read any input from stdin/console.
Output Format
Compare the two linked lists and return 1 if the lists are equal. Otherwise, return 0. Do NOT print anything to stdout/console.
Sample Input
NULL, 1 --> NULL
1 --> 2 --> NULL, 1 --> 2 --> NULL
Sample Output
0 1 Explanation 1. We compare an empty list with a list containing 1. They don't match, hence return 0. 2. We have 2 similar lists. Hence return 1. int CompareLists(Node *headA, Node* headB) { int flag=0; // This is a "method-only" submission. // You only need to complete this method while(headA!=NULL) { if(headA->data==headB->data) { flag=1; } else { flag=0; break; } headA=headA->next; headB=headB->next; } if(headB!=NULL) { flag=0; } return flag; }Note that this approach is not only specific to this type of question you can use this sorting technique in various questions such as if you want to check whether to word are anagrams of one another or not. You can simply sort them and compare them to find out. This approach is also helpful in other questions so i suggest that its better to learn this tricks and keep in mind while attempting the question you may find it useful. You can always search for more coding problems like this to get comfortable to this to approach or you can find new problems where this approach is applicable. So go and search more problems like this and check whether this approach is useful or not and you can tell me about it by commenting and writing about this to me.
Try more and more problems that you find on string and arrays and try to develop an approach the will help you understand problems rather then learning the solution. Once you know how to approach the problem you will able to solve any problem related to strings and array. I wish best of luck to you for your future.